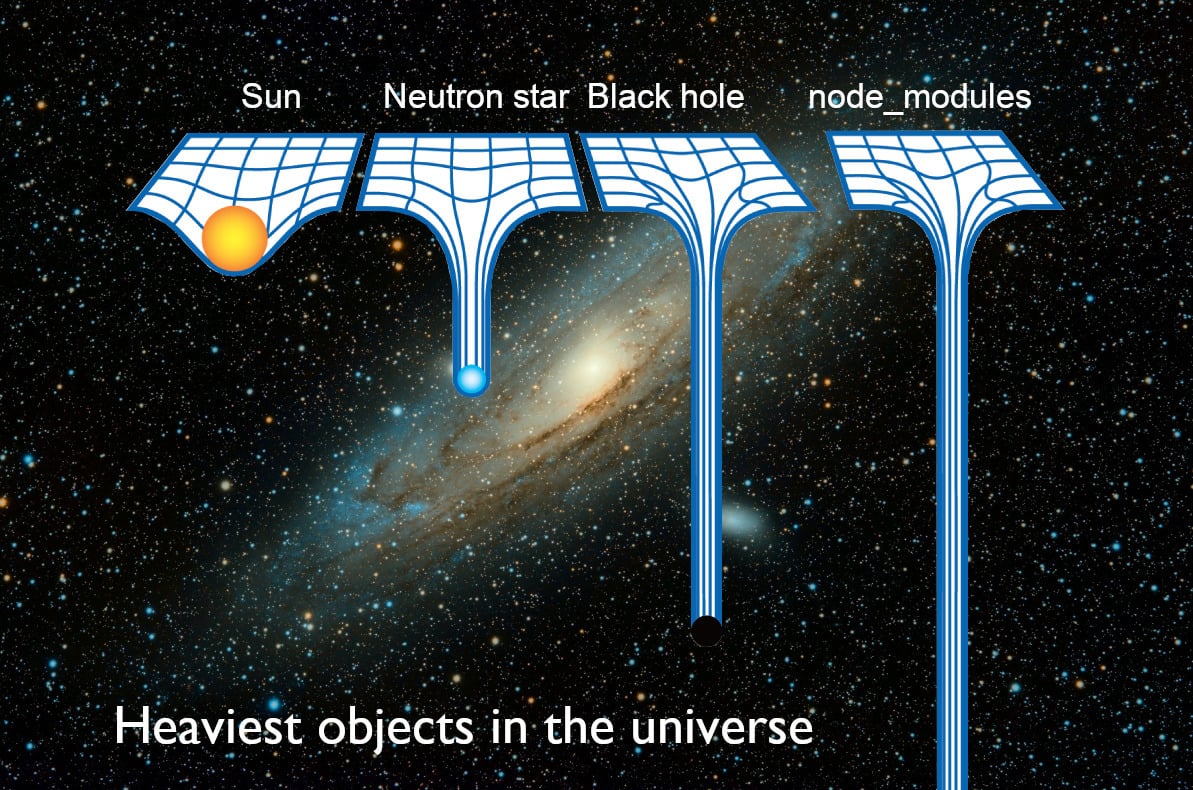
Why Are Node.js Modules So Large for Simple Projects? A Deep Dive into the Node.js Ecosystem
When you start a simple project with Node.js, you might notice something unexpected: your node_modules folder quickly balloons in size, even for the most basic tasks. You might wonder, “Why does my small project need so many modules?” You’re not alone. Many developers ask this question, especially when the size of Node.js modules becomes a burden for version control or deployment. Let’s dig into the core reasons why Node.js modules get so large and why that’s not always a bad thing.
The Ecosystem of Node.js Modules: A Story of Exponential Growth
Node.js has an ecosystem that’s both powerful and modular, but this comes at a price. What might seem like a simple task often pulls in dozens or even hundreds of dependencies. Here’s a detailed breakdown of the factors contributing to this complexity.
1. Dependency Chains and Transitive Dependencies
The biggest reason for the bloat in your node_modules folder is dependency chains. When you install a package like express, you’re not just getting Express itself. You’re getting everything that Express relies on to work properly, and then everything those dependencies rely on as well. This creates a chain of dependencies, known as transitive dependencies.
Imagine it like this: You want to build a simple house, but every tool you use brings its own set of helpers. Those helpers bring their own tools, and before you know it, your toolbox is overflowing!
2. The Culture of Micro-Packages
In the Node.js world, developers have adopted a philosophy of building small, focused modules that do one thing well. It’s not uncommon to see packages that perform basic tasks like checking if a variable is a string or converting text to uppercase. While this modularity is great for code reusability, it means that even small projects can pull in hundreds of tiny packages.
Why should you care?
- Pros: Easier code management, more reusable components.
- Cons: Increased node_modules size, dependency management complexity.
3. Development and Production Code in One
Node.js packages often come bundled with both development tools and production-ready code. This means that when you install a package, you’re getting everything: tests, documentation, build tools, examples, and more.
While these files don’t affect your app in production, they still occupy space in the node_modules folder during development. This is a crucial reason why your project might seem unnecessarily large even when you’re only using a fraction of the code.
4. Backward Compatibility and Polyfills
To maintain compatibility with different environments and older Node.js versions, many packages include polyfills or compatibility code. This ensures that your app runs smoothly across all scenarios but also adds a significant amount of code that might be unnecessary for your specific use case.
Why should you care?
- Compatibility is good, but it comes at the cost of larger package sizes.
- If you’re targeting a specific Node.js version, you might be pulling in more code than you need.
5. Tooling Overload: Modern Development Complexity
Modern JavaScript development involves tools like Webpack, Babel, and ESLint to handle build processes, linting, and code transformations. Each of these tools brings in a massive number of dependencies, even if you’re building a relatively simple project.
While these tools help in optimizing the code for production and adding advanced features, they also add significantly to the size of your development environment.
6. Multiple Versions of the Same Package
Due to Node.js’s flexible package management system, it’s common to find multiple versions of the same dependency in your project. If two different libraries require different versions of the same module, npm installs both to avoid compatibility issues. This practice leads to module duplication, which can quickly inflate your project size.
7. Dead Code and Unused Features
Not every piece of code in your node_modules is used in your project. Many packages include features that you might never use, known as dead code. While tools like Webpack can strip out unused code in production, it still remains in your node_modules folder, making it larger than necessary during development.
How to Tame the node_modules Beast
Now that we know the reasons why Node.js modules are so large, here are some practical tips to manage the size of your project:
- Use Production Installations: Use npm install –production or NODE_ENV=production npm install to skip development dependencies in production environments.
- Bundle Your Code: Use tools like Webpack or Rollup to bundle your JavaScript files and reduce the overall size of your deployment.
- Remove Unused Packages: Regularly clean up your node_modules using commands like npm prune or delete it entirely and reinstall with only the necessary dependencies.
- Choose Lightweight Alternatives: Where possible, use lightweight libraries instead of full-featured frameworks. For instance, use dayjs instead of moment, or native fetch instead of axios.
Conclusion: The Price of Modularity and Flexibility
Node.js’s massive module size for simple projects is not just a flaw; it’s a byproduct of a highly modular, flexible ecosystem. While it might seem like overkill, this approach allows developers to build scalable, maintainable applications by using small, single-purpose packages. The trade-off is the size, but the benefit is in the flexibility and reusability of the code.
Keywords and Hashtags
Keywords: Node.js modules, npm packages, dependency management, JavaScript modules, Node.js project size, package bloat, modular design, transitive dependencies, npm ecosystem, Node.js development.
Hashtags: #NodeJS #npm #JavaScript #WebDevelopment #CodingTips #NodeModules #DependencyManagement #SoftwareDevelopment #ModularDesign #Programming
Table of Contents
- The Ecosystem of Node.js Modules: A Story of Exponential Growth
- 1. Dependency Chains and Transitive Dependencies
- 2. The Culture of Micro-Packages
- 3. Development and Production Code in One
- 4. Backward Compatibility and Polyfills
- 5. Tooling Overload: Modern Development Complexity
- 6. Multiple Versions of the Same Package
- 7. Dead Code and Unused Features
- How to Tame the node_modules Beast
- Conclusion: The Price of Modularity and Flexibility
Leave a Reply